Speech Synthesizer Python
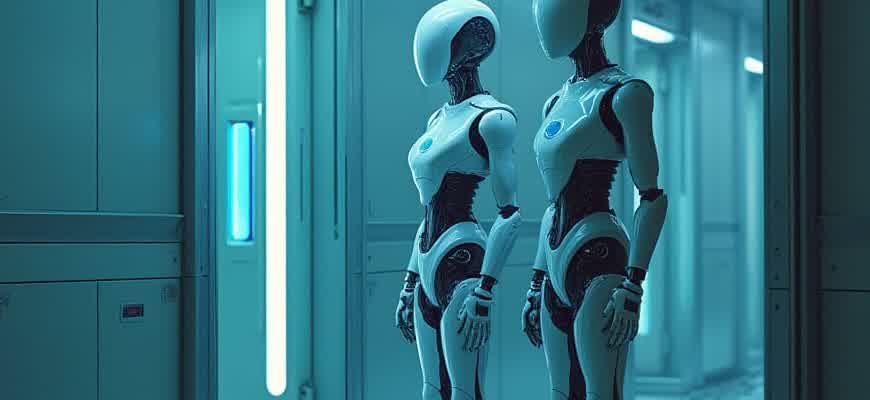
Python offers powerful tools for creating speech synthesizers, allowing developers to convert text into natural-sounding audio. By utilizing various libraries, one can easily integrate speech generation into applications, enhancing user experience.
One of the most widely used libraries for this purpose is pyttsx3, which works offline and supports multiple speech engines. It is cross-platform, making it suitable for a variety of environments.
Note: pyttsx3 supports both male and female voices and can adjust speech rate and volume.
To get started with speech synthesis in Python, follow these steps:
- Install pyttsx3 library:
pip install pyttsx3
- Import the library in your script:
import pyttsx3
- Initialize the engine and configure properties such as rate and volume.
- Use the engine to say the desired text:
engine.say("Hello World")
Additionally, there are other libraries like gTTS (Google Text-to-Speech) that require an internet connection but offer high-quality voice output.
Library | Offline Support | Voice Quality |
---|---|---|
pyttsx3 | Yes | Good |
gTTS | No | Excellent |
How to Leverage Python for Speech Synthesis: A Practical Guide
Python offers powerful libraries for creating speech synthesis applications, making it an accessible tool for developers and researchers alike. With Python, you can generate high-quality speech from text, opening possibilities for voice assistants, accessibility tools, and interactive systems. The flexibility of Python’s libraries, such as pyttsx3, gTTS, and speech_recognition, allows you to choose the right tool for your needs and even integrate speech capabilities into larger applications.
This guide covers the essential steps to start building speech synthesis applications using Python. It focuses on practical libraries and their implementation, along with key considerations when working with audio processing in real-world projects.
Setting Up Your Speech Synthesis Environment
Before diving into coding, you'll need to install the necessary libraries. Here's how you can set up a simple Python environment for speech synthesis:
- Install the required libraries with pip:
pip install pyttsx3 gTTS
- Verify the installation by importing the libraries in a Python script.
Using pyttsx3 for Offline Speech Synthesis
pyttsx3 is a popular library for offline speech synthesis, meaning it doesn't require an internet connection. It's compatible with both Windows and Linux platforms. Here's a simple example to generate speech:
import pyttsx3 engine = pyttsx3.init() engine.say("Hello, welcome to Python speech synthesis!") engine.runAndWait()
In this example, the say function converts the text into speech, while runAndWait processes the speech request.
Exploring gTTS for Online Speech Synthesis
If you're open to using an online service, the Google Text-to-Speech (gTTS) library is a great choice. It offers high-quality voices powered by Google's Text-to-Speech API.
from gtts import gTTS import os tts = gTTS(text="Hello, this is an example of gTTS in action", lang='en') tts.save("example.mp3") os.system("start example.mp3")
Here, gTTS generates an MP3 file, and the system command plays the audio file. It's important to note that using gTTS requires an active internet connection for text-to-speech conversion.
Key Considerations for Speech Synthesis Projects
Tip: Ensure that you handle the conversion speed and voice modulation to provide a natural listening experience. Test with different voices and speeds for optimal user interaction.
When integrating speech synthesis into an application, consider these key factors:
- Voice Selection: Both pyttsx3 and gTTS offer multiple voices and languages, allowing for a wide range of customization. Choose the one that best suits your target audience.
- Speed Control: Speed and pitch adjustments are crucial for a more human-like experience. Experiment with these features to improve speech clarity.
- Error Handling: Ensure that your code includes error handling, particularly for network issues when using online APIs like gTTS.
Conclusion
By using libraries like pyttsx3 and gTTS, Python developers can create versatile speech synthesis applications for various use cases. Whether you need offline speech capabilities or online-powered high-quality synthesis, these tools are easy to implement and offer substantial flexibility for customization.
Integrating Speech Synthesis Libraries in Python Projects
Speech synthesis is becoming an essential tool for enhancing the accessibility and interactivity of applications. Python, being a versatile language, offers several libraries for speech synthesis, allowing developers to integrate vocal feedback into their projects with ease. This capability is particularly useful for applications in education, accessibility, and virtual assistants, where auditory output is required.
Incorporating speech synthesis into a project involves selecting the appropriate library, configuring it according to your needs, and implementing the necessary functions. Below are some of the most popular Python libraries that can be integrated into your projects for speech synthesis.
Common Python Speech Synthesis Libraries
- pyttsx3: A text-to-speech conversion library that works offline and supports multiple platforms (Windows, Mac, Linux).
- gTTS (Google Text-to-Speech): An easy-to-use library for converting text into speech using Google’s Text-to-Speech API. Requires an internet connection.
- SpeechRecognition: Mainly used for speech-to-text but can also be combined with TTS libraries for complete speech-driven applications.
Basic Integration Steps
- Install the library: Start by installing the required library using pip, for example,
pip install pyttsx3
. - Initialize the engine: For most libraries like pyttsx3, you need to initialize the speech engine before using it.
- Convert text to speech: Use the library’s methods to convert text into spoken output.
- Configure settings: Customize speech rate, volume, and voice type to match your project’s requirements.
Note: Always check for platform compatibility when selecting a speech synthesis library. Some libraries may perform better on specific operating systems or devices.
Example Code with pyttsx3
Step | Code |
---|---|
Install the library | pip install pyttsx3 |
Initialize the engine | import pyttsx3 |
Convert text to speech | engine.say("Hello, world!") |
Customize the voice | voices = engine.getProperty('voices') |
Choosing the Optimal Speech Synthesis Library for Python
When integrating text-to-speech functionality into your Python application, selecting the appropriate speech synthesis library is crucial for achieving high-quality and efficient results. The Python ecosystem offers various libraries, each catering to different use cases and system configurations. Factors such as ease of use, available voices, platform compatibility, and customization options play a significant role in the decision-making process. Below, we explore some of the most popular libraries for speech synthesis in Python and their key characteristics.
Before diving into specific libraries, it's important to evaluate your needs. Are you focusing on simplicity, or do you require more advanced features like multilingual support and fine-grained control over speech parameters? Additionally, consider the library's community support and documentation, as these elements can significantly affect your development process.
Popular Speech Synthesis Libraries in Python
- gTTS (Google Text-to-Speech): A simple library that connects to Google's TTS API. It is quick to set up, offers multiple languages, and generates high-quality speech. However, it requires an internet connection and may not provide deep customization.
- pyttsx3: A cross-platform offline library that supports both Windows and Linux systems. Unlike gTTS, pyttsx3 does not rely on internet access and can offer more customization, though it may not produce voices as natural as Google's offering.
- SpeechRecognition: Primarily used for speech recognition, it can also handle speech synthesis with limited features. It’s best suited for applications where both recognition and synthesis are required.
Key Factors to Consider
- Ease of Use: Some libraries are easier to implement, especially for simple applications. For example, gTTS has a minimal setup, making it perfect for quick integration.
- Voice Quality: If natural-sounding voices are a priority, libraries like pyttsx3 may offer more flexibility, although they may not achieve the same level of quality as cloud-based services.
- Platform Support: Ensure the library you choose is compatible with your target operating systems. For instance, pyttsx3 works well on both Windows and Linux, while gTTS is web-based and works anywhere with an internet connection.
- Customization: If fine control over pitch, speed, and volume is essential, libraries like pyttsx3 provide more advanced options.
Important: Consider whether offline functionality is critical for your application. While online services offer better voice quality, they may not be suitable for scenarios with limited or no internet access.
Comparative Overview
Library | Offline Support | Customization | Platform Compatibility |
---|---|---|---|
gTTS | No | Basic | Cross-platform |
pyttsx3 | Yes | High | Windows, Linux |
SpeechRecognition | Partial | Moderate | Cross-platform |
How to Convert Text to Speech Using Python: A Simple Guide
Converting text to speech (TTS) in Python is a straightforward task, thanks to several libraries that make this process easy and efficient. One of the most popular libraries for this purpose is pyttsx3, which works offline and is compatible with both Windows and Linux. To begin with, you need to install this package and write a few lines of code to start converting text into speech.
Once the setup is done, you can customize your TTS application to suit your needs, such as adjusting the speech rate, volume, or voice type. The entire process involves simple steps and doesn’t require deep knowledge of audio processing or complex algorithms.
Steps to Convert Text to Speech in Python
- Install the Required Library
- Run the command
pip install pyttsx3
in your terminal to install the pyttsx3 library.
- Run the command
- Initialize the TTS Engine
- Import pyttsx3 and initialize the engine by using
engine = pyttsx3.init()
.
- Import pyttsx3 and initialize the engine by using
- Set Parameters (Optional)
- You can adjust properties such as rate (speed of speech), volume, and voice by using
engine.setProperty('rate', 150)
,engine.setProperty('volume', 1)
, andengine.setProperty('voice', voice.id)
respectively.
- You can adjust properties such as rate (speed of speech), volume, and voice by using
- Convert Text to Speech
- Use
engine.say('Your text here')
to convert your input text to speech. - To play the speech, use
engine.runAndWait()
.
- Use
Tip: Ensure your device has speakers or a proper audio output device connected to hear the speech correctly.
Example Code
Step | Code |
---|---|
Install Library | pip install pyttsx3 |
Initialize Engine | engine = pyttsx3.init() |
Set Properties | engine.setProperty('rate', 150) |
Convert Text | engine.say('Hello, this is a text-to-speech example.') |
Play Speech | engine.runAndWait() |
Fine-Tuning Voice Parameters for Realistic Audio Output in Python
Creating a natural-sounding voice using a speech synthesis tool in Python requires careful adjustment of several parameters. These parameters, which influence the pitch, speed, tone, and timbre of the voice, are essential in producing an output that mimics human speech as closely as possible. In this context, achieving a realistic audio output involves modifying various settings to match the desired vocal characteristics.
One of the most important aspects of voice synthesis is balancing the pace and smoothness of speech. Too fast a pace can make the voice sound robotic, while a too-slow pace can create unnatural pauses. By adjusting voice characteristics like pitch and speed, one can generate audio that feels more conversational and engaging.
Key Voice Parameters to Fine-Tune
- Pitch: Controls the perceived frequency of the voice. A higher pitch can create a more energetic tone, while a lower pitch conveys a calmer, deeper sound.
- Rate: Refers to the speed at which the speech is delivered. A natural range usually falls between 150-200 words per minute.
- Volume: Adjusting the volume ensures the voice is clear without being overwhelming. Proper volume levels contribute to the realism of the output.
- Voice Timbre: Defines the unique texture of the voice, affecting whether the output sounds more robotic or human-like.
- Pauses: Adding appropriate pauses between phrases or words can create a more natural flow of speech.
Parameter Adjustment Example
- First, select the speech synthesis engine, such as gTTS or pyttsx3.
- Adjust the rate using the `rate` parameter to set a comfortable speaking speed.
- Modify the pitch by adjusting the `pitch` value in your synthesizer's settings.
- Ensure proper pauses are inserted with the `pause` function or by using custom punctuation in the input text.
Table: Example of Adjusting Parameters
Parameter | Default Value | Adjusted Value |
---|---|---|
Pitch | 50 | 80 (higher pitch) |
Rate | 150 words/min | 180 words/min |
Volume | 1.0 | 0.9 |
Fine-tuning these parameters can significantly enhance the quality of your synthetic voice, making it sound more natural and engaging for listeners.
Optimizing Speech Synthesis for Large-Scale Deployments
When implementing speech synthesis systems in large-scale applications, performance optimization becomes a crucial aspect of the process. The need for fast, accurate, and resource-efficient voice generation is especially vital when handling thousands of requests per second. A variety of factors, including model architecture, input preprocessing, and output postprocessing, can significantly impact system performance. Addressing these areas is essential for ensuring smooth operation, minimal latency, and scalability across diverse use cases.
In this context, optimization strategies typically involve a combination of algorithmic improvements, hardware enhancements, and efficient data management techniques. Techniques like model compression, batching of inference tasks, and intelligent resource allocation can help in achieving the necessary throughput. Below, we outline key considerations for optimizing speech synthesis systems at scale.
Key Optimization Techniques
- Model Compression: Reducing the size of the neural network models without sacrificing too much accuracy can drastically improve inference speed. Techniques like quantization and pruning can be employed.
- Batch Processing: Instead of processing requests individually, batching multiple requests together allows for more efficient use of hardware resources and can speed up the overall process.
- Optimized Data Pipeline: Implementing an optimized data flow from input processing to synthesis output helps in reducing the time spent on unnecessary steps, enabling quicker responses.
Performance Metrics
Performance measurement is crucial for understanding the efficiency of the speech synthesis system. Common metrics include:
Metric | Description |
---|---|
Latency | Time taken for a speech synthesis request to be processed and output generated. |
Throughput | The number of synthesis tasks handled per unit of time. |
Accuracy | How closely the generated speech matches the intended natural sound quality and pronunciation. |
“To achieve the best performance in large-scale speech synthesis, a balance between model accuracy and computational efficiency must be struck.”
Recommended Strategies for Large-Scale Systems
- Utilizing Specialized Hardware: Deploying on GPU or specialized accelerators like TPUs can significantly reduce the time needed for processing individual requests.
- Edge Computing: Offloading some processing tasks to edge devices can reduce the load on centralized servers and improve response time.
- Adaptive Scaling: Implementing a system that dynamically adjusts resources based on demand ensures that the system can handle fluctuating loads efficiently.
Troubleshooting Common Issues in Python Speech Synthesis
Working with speech synthesis in Python can be a powerful way to create interactive applications. However, users may encounter various challenges while trying to integrate text-to-speech (TTS) functionality. Understanding the root causes of common issues can help in quickly resolving them and ensuring smooth operation.
This guide covers some of the most frequently encountered problems, providing actionable solutions. The following sections outline typical errors, their causes, and how to address them effectively when using speech synthesis libraries in Python.
1. No Sound Output
One of the most common issues users face is the lack of sound when executing a speech synthesis program. This can be caused by several factors, such as incorrect audio settings, missing dependencies, or misconfigurations in the TTS library.
- Check Audio Device: Ensure the correct audio output device is selected in your system settings.
- Verify Dependencies: Libraries like pyttsx3 or gTTS require specific backend engines (e.g., SAPI5 or espeak). Make sure these are properly installed.
- Update Library: Sometimes, simply updating the TTS library to the latest version can resolve sound-related issues.
Note: Ensure that your system's audio is not muted, and check the volume settings before troubleshooting software-related issues.
2. Poor Quality or Distorted Speech
When speech synthesis results in poor-quality or distorted audio, it is typically related to the voice engine or system settings. Adjusting these elements can significantly improve the output.
- Change Voice Settings: Some speech engines allow switching between different voices (e.g., male, female, different accents). Experiment with these settings to improve speech clarity.
- Check Engine Configuration: For engines like pyttsx3, make sure the correct rate and volume are configured. Use the following commands:
Setting | Command |
---|---|
Speech Rate | engine.setProperty('rate', 150) |
Volume | engine.setProperty('volume', 1.0) |
Voice | engine.setProperty('voice', voices[0].id) |
3. Library-Specific Errors
Sometimes, specific errors can arise due to the choice of library. Each TTS library has its own set of potential issues and solutions.
- pyttsx3: Engine Not Found – This error occurs when the backend engine is unavailable. Reinstalling or switching to a different engine may resolve the issue.
- gTTS: Network Issues – gTTS requires an internet connection. If network connectivity is unstable, it may cause failures in generating speech.
Enhancing User Interaction with Speech Synthesis in Python-Based Apps
Speech synthesis technology has evolved significantly, offering a variety of tools to enhance user experiences in applications. Python provides several libraries for incorporating text-to-speech (TTS) functionality, allowing developers to create more accessible, interactive, and dynamic user interfaces. By integrating voice output into an application, developers can create a more engaging and intuitive experience, especially for users with disabilities or those who prefer voice interaction over text-based input.
By leveraging speech synthesis, developers can build more immersive environments where users can interact with the app through voice commands, receive spoken feedback, or enjoy an overall richer interaction. Libraries such as pyttsx3, gTTS, and SpeechRecognition offer various features, each catering to different needs and providing flexibility when designing voice-driven apps.
Key Benefits of Speech Synthesis in Python Apps
- Enhanced Accessibility: Speech synthesis helps make applications more accessible to users with visual impairments or reading difficulties.
- Increased Engagement: Spoken responses can lead to more immersive experiences, improving overall user engagement.
- Hands-Free Interaction: Users can interact with applications without needing to touch or type, providing convenience and multitasking opportunities.
Implementing Speech Synthesis with Python
- Choosing the Right Library: Select a Python package that suits the needs of your application. Popular choices include pyttsx3 for offline synthesis and gTTS for Google-powered voice output.
- Customizing Speech Output: Adjust the voice speed, pitch, and volume for optimal user experience. Most libraries provide these options for fine-tuning.
- Integrating with User Inputs: Combine speech synthesis with voice recognition to create interactive voice-enabled systems.
Integrating speech synthesis not only improves accessibility but also makes it possible for applications to reach a wider audience, particularly those with motor disabilities or older adults who may find typing challenging.
Speech Synthesis Features Comparison
Library | Offline Support | Voice Customization | Supported Platforms |
---|---|---|---|
pyttsx3 | Yes | Speed, Volume, Pitch | Windows, macOS, Linux |
gTTS | No | Voice, Language | Windows, macOS, Linux (Requires Internet) |
SpeechRecognition | No | None | Windows, macOS, Linux (Requires Internet) |