Arduino Ai Voice Assistant
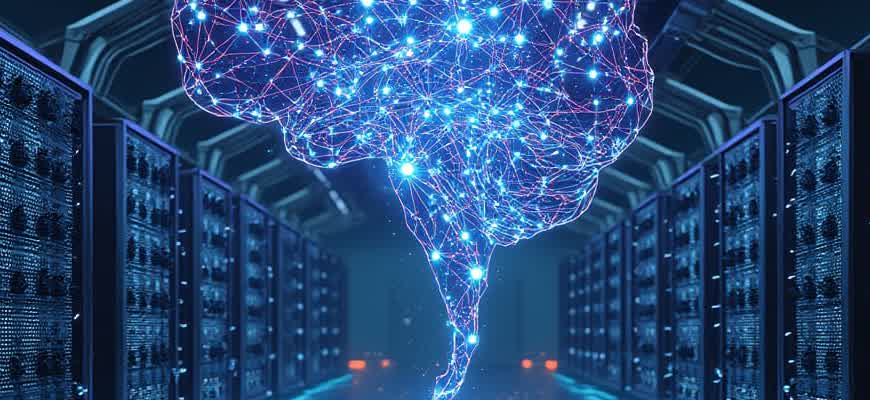
The development of a voice-controlled assistant using Arduino involves integrating various components, including a microphone, speaker, and an AI processing unit. Arduino, a versatile microcontroller platform, serves as the base for hardware interaction, while external modules are used to process voice input and generate responses.
Key components involved in the project include:
- Arduino board (e.g., Arduino Uno, Nano)
- Microphone or sound sensor
- Speaker for audio output
- AI processing module (such as a Raspberry Pi or cloud-based services)
- Wi-Fi or Bluetooth for communication
Important:
Voice recognition and processing require significant computational power, often handled by more advanced systems like Raspberry Pi, with Arduino acting as an intermediary for hardware control.
Here is a general overview of the process:
- Capture audio input from the user through the microphone.
- Send the audio signal to an AI system for processing and understanding.
- Generate a suitable response based on the processed data.
- Output the response through the speaker.
Table: Components for Voice Assistant
Component | Description | Example |
---|---|---|
Arduino Board | Microcontroller for interfacing with hardware | Arduino Uno, Nano |
Microphone | Captures sound for voice input | Electret Mic |
Speaker | Outputs voice responses | Small speaker, buzzer |
AI Processor | Processes voice data and generates responses | Raspberry Pi, cloud-based service |
Integrating Arduino with Popular Voice Recognition APIs
Connecting an Arduino platform with popular voice recognition APIs opens up a range of possibilities for creating interactive and automated systems. By using cloud-based or local voice recognition technologies, developers can harness voice commands to control physical devices or gather information from the Arduino. APIs like Google Speech-to-Text, IBM Watson, and Microsoft Azure provide reliable and flexible tools to process speech data, and integrating these with Arduino can be done through various methods, such as Bluetooth or Wi-Fi modules like ESP8266 or ESP32.
In this guide, we'll explore how to set up an Arduino project with voice recognition, outlining the basic steps and tools needed for successful integration. The process involves preparing the hardware, connecting to the API via a network module, and handling the received voice data to trigger actions on your Arduino board. Here's how to do it:
1. Prepare the Arduino Hardware
- Choose an Arduino board (e.g., Arduino Uno, Mega, or any model compatible with your project).
- Integrate a network module (Wi-Fi or Bluetooth) such as ESP8266 or ESP32.
- Ensure you have a microphone module (e.g., Elechouse Voice Recognition Module or any other compatible sensor).
- Connect the Arduino to a power source and ensure all components are properly wired.
2. Set Up Voice Recognition API
- Sign up for a voice recognition API service (Google, IBM Watson, or Microsoft Azure).
- Get the necessary API keys and access tokens for communication between your Arduino and the API.
- Use a library like WiFi.h or ESP8266WiFi.h to establish a connection to the internet.
- Write a program on Arduino that sends audio data (from the microphone) to the cloud API.
3. Handling API Responses
The next step is to process the response from the API. After the voice data is sent, the API will return text commands. The Arduino can then parse this data and trigger corresponding actions. For example, a command like "turn on the light" will activate an LED connected to one of the Arduino’s pins. Here's a simple example of an API response handling structure:
Voice Command | Arduino Action |
---|---|
Turn on light | Activate LED connected to pin 13 |
Play music | Send signal to audio module to play predefined file |
Set alarm | Activate a buzzer for a set duration |
Note: Ensure that the response time from the API is within the acceptable range for real-time interaction, as some services may introduce latency.
4. Testing and Debugging
Once your system is set up, perform thorough testing by giving different voice commands and observing how the Arduino responds. Debugging may involve checking network connectivity, verifying API keys, and troubleshooting hardware connections.
Creating Personalized Voice Commands for Home Automation
Custom voice commands provide an intuitive way to interact with home automation systems. By tailoring these commands to specific needs, users can streamline everyday tasks such as controlling lights, adjusting temperatures, and managing appliances. Arduino-based voice assistants, combined with speech recognition software, offer an affordable and flexible platform to build and implement such custom commands.
The process of designing and integrating voice commands begins with defining the automation goals. Depending on the home setup, the voice commands could range from simple tasks, like turning on lights, to more complex functions, such as controlling the home security system or setting up a custom morning routine.
Steps to Build Custom Commands
- Define the Commands: Determine the specific actions you want to control (e.g., lighting, heating, security). Write down simple phrases that can be easily recognized by the voice assistant.
- Integrate with Arduino: Connect sensors or relays to your Arduino board to control the hardware. Use a microphone module or Bluetooth for receiving voice input.
- Programming Speech Recognition: Use speech-to-text software (e.g., Google Speech API or CMU Sphinx) to convert voice input into readable commands for Arduino.
- Test and Optimize: Once the commands are configured, test the system with various voice samples to ensure accurate recognition. Optimize for different accents and noise levels.
Examples of Common Voice Commands
Command | Action |
---|---|
"Turn on the lights" | Activates the light system connected to Arduino |
"Set the temperature to 22°C" | Adjusts the thermostat to the specified value |
"Lock the front door" | Triggers a relay to activate the door lock mechanism |
Important: Ensure that your voice assistant system can handle background noise and different pronunciations for better performance and reliability.
Considerations for Advanced Integration
- Security: Be cautious about the security implications of voice-controlled systems, especially with commands related to locking doors or controlling alarm systems.
- Compatibility: Ensure that all devices in your home automation setup are compatible with Arduino and the voice recognition software you plan to use.
- Expanding Functionality: Over time, you can add more advanced commands, such as scheduling routines or interacting with other smart home ecosystems like Google Home or Amazon Alexa.
Optimizing Voice Recognition for Accuracy in Noisy Environments
Voice recognition systems, especially those used in embedded projects like Arduino-powered AI assistants, face significant challenges when it comes to accurately interpreting speech in environments with high levels of ambient noise. For these systems to function properly, they must not only detect speech clearly but also filter out irrelevant background sounds that can distort or interfere with voice commands.
To achieve better accuracy in noisy environments, engineers can implement various techniques that enhance speech clarity and suppress unwanted noise. These strategies include advanced algorithms for noise reduction, the use of specialized microphones, and the integration of machine learning models trained to distinguish speech from background noise.
Methods for Enhancing Speech Recognition
- Noise Suppression Algorithms: Algorithms such as spectral subtraction and Wiener filtering help minimize the impact of background noise by isolating the human voice.
- Directional Microphones: These microphones are designed to capture sound from a specific direction, improving the signal-to-noise ratio.
- Machine Learning Models: Custom AI models can be trained to recognize and ignore specific types of noise, learning to identify speech patterns even in challenging conditions.
- Echo Cancellation: Removing echoes caused by surrounding surfaces helps prevent sound reflections that could interfere with voice recognition.
Effective noise cancellation not only improves speech recognition but also reduces power consumption, making it ideal for portable Arduino devices.
Important Considerations
Technique | Benefit | Limitation |
---|---|---|
Noise Suppression | Reduces interference from ambient noise | Can distort speech if over-applied |
Directional Microphones | Improves voice capture in noisy environments | Limited to specific angles, may not work in all conditions |
Machine Learning Models | Improves recognition by learning noise patterns | Requires substantial data for training |
Strategies for Reducing False Positives
- Voice Activity Detection (VAD): By determining whether a signal contains human speech or not, VAD helps the system ignore irrelevant sounds like wind or machinery.
- Noise Profile Adjustment: Periodically updating the noise profile based on environmental changes ensures continuous accuracy.
- Multiple Microphone Setup: Using multiple microphones placed at different locations allows the system to triangulate sound sources and enhance speech clarity.
Building a Custom IoT Assistant with Arduino
Creating a personal assistant for IoT devices using Arduino is an exciting and practical way to integrate voice control and automation into your smart home ecosystem. With the combination of affordable hardware, open-source software, and voice recognition technologies, Arduino provides a flexible platform to create custom solutions tailored to specific needs. Whether you're controlling lights, temperature, or any other IoT device, a voice-based assistant can streamline everyday tasks and improve accessibility.
To build a functional IoT assistant, you’ll need to connect various components and integrate voice recognition software. Arduino acts as the central hub, interfacing with sensors, actuators, and other IoT devices. By combining Arduino with voice modules like the Alexa or Google Assistant SDK, or even integrating open-source libraries, you can create a seamless communication link between the user and the connected devices.
Key Components Needed for the Project
- Arduino Board - The brain of your system, capable of managing I/O operations.
- Microphone Module - Captures voice input from the user.
- Voice Recognition Software - Analyzes and processes speech to control devices.
- Wi-Fi/Bluetooth Module - Enables wireless communication with other devices.
- IoT Devices - Any smart device (lights, thermostat, etc.) to be controlled.
Steps to Create a Voice-Controlled IoT Assistant
- Set up the Arduino board with the necessary voice recognition software.
- Connect the microphone module to the Arduino board to capture voice input.
- Program the system to recognize specific voice commands.
- Link IoT devices to the Arduino board using a Wi-Fi or Bluetooth module.
- Test the system to ensure reliable voice control of the IoT devices.
Example Wiring Setup
Component | Connection |
---|---|
Microphone | Connect to Arduino analog input pin |
Wi-Fi Module (e.g., ESP8266) | Connect to Arduino via Serial |
Relay/Actuators | Connect to digital I/O pins on Arduino |
Tip: Start with a simple command like turning a light on or off before expanding to more complex tasks like temperature control or multi-device management.
Voice Command-based Control of Arduino Projects: Practical Examples
Integrating voice commands into Arduino projects opens up a new realm of possibilities, allowing users to interact with their creations using natural language. Voice-controlled systems can be implemented through various components, including microphones, speech recognition modules, and digital assistants like Google Assistant or Alexa. These projects not only enhance user experience but also add a layer of convenience and accessibility to devices. In this context, understanding how voice commands can trigger actions in Arduino projects becomes essential for anyone interested in building advanced and user-friendly devices.
Voice command-based control can be applied to a variety of practical Arduino projects, such as home automation systems, robotic assistants, or even simple devices like lights and fans. These systems typically rely on an intermediary platform that translates voice inputs into commands that the Arduino can understand and execute. Below, we will explore some examples of how these setups can be practically implemented, ranging from basic switches to more complex robotic tasks.
Practical Examples of Voice-Controlled Arduino Projects
Below are a few practical examples of how voice commands can control different aspects of Arduino-based projects:
- Voice-Controlled Home Automation: Control home appliances like lights, fans, or air conditioners through voice commands. This can be achieved using a microphone module, a speech recognition platform, and an Arduino to interface with the electrical devices.
- Voice-Activated Robot: In this project, users can issue commands like "move forward", "turn left", or "stop", and the robot will respond accordingly. A speech-to-text system processes the commands, sending appropriate signals to the Arduino to control the robot's motors.
- Voice-Based Smart Lock: Voice commands can be used to unlock doors or activate security systems. For instance, a phrase like "open door" can trigger the servo motor attached to the lock mechanism.
Voice Command Control Workflow
The general workflow for implementing voice commands in Arduino projects follows these steps:
- Voice Input: The microphone or a smart assistant captures the voice command.
- Speech Recognition: The voice command is processed using a speech recognition module or cloud-based service (like Google Speech API or Amazon Alexa). This module converts the spoken word into a digital signal.
- Signal Processing: The Arduino receives the processed command and translates it into an action, such as turning on a light or moving a motor.
- Execution: The connected hardware executes the desired function based on the recognized voice command.
Example of a Simple Voice-Controlled Light System
In the following table, a basic voice-controlled light system is outlined:
Step | Action | Components Used |
---|---|---|
1 | Voice command "Turn on the light" | Microphone, Speech Recognition Module |
2 | Signal sent to Arduino for processing | Arduino, Relay |
3 | Relay switches the light on | Relay, Light Bulb |
Tip: To improve accuracy, it's important to use a high-quality microphone and minimize background noise when implementing voice recognition in your Arduino projects.