Google Cloud Text to Speech Api Example
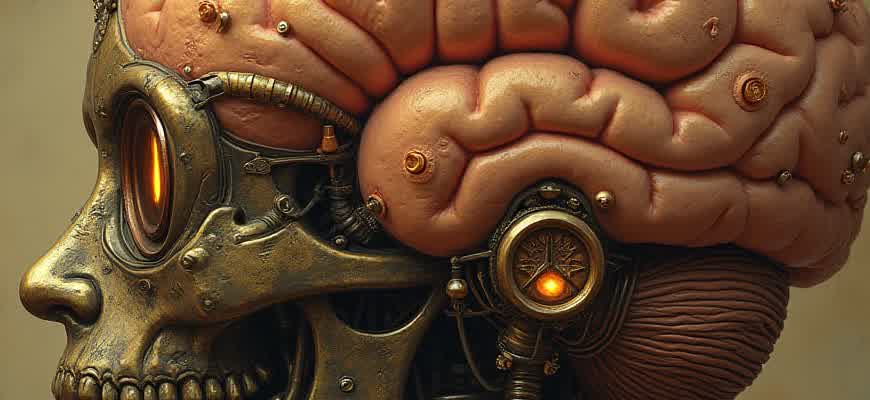
Google Cloud Text-to-Speech API allows developers to convert text into natural-sounding speech using Google's advanced machine learning models. With this API, users can generate audio from text in multiple languages, offering high-quality voices for various applications such as virtual assistants and accessibility tools.
Getting Started
- Set up a Google Cloud project and enable the Text-to-Speech API.
- Authenticate using a service account key or OAuth 2.0 credentials.
- Install the Google Cloud SDK or use client libraries for integration with your application.
Code Example
Here’s a simple example in Python to demonstrate how to use the API:
from google.cloud import texttospeech client = texttospeech.TextToSpeechClient() input_text = texttospeech.SynthesisInput(text="Hello, world!") voice = texttospeech.VoiceSelectionParams( language_code="en-US", ssml_gender=texttospeech.SsmlVoiceGender.NEUTRAL ) audio_config = texttospeech.AudioConfig(audio_encoding=texttospeech.AudioEncoding.MP3) response = client.synthesize_speech(input=input_text, voice=voice, audio_config=audio_config) with open("output.mp3", "wb") as out: out.write(response.audio_content)
Key API Features
Feature | Description |
---|---|
Language Support | Supports over 30 languages and dialects. |
Voice Selection | Offers various voice types such as male, female, and neutral. |
Audio Formats | Generates audio in MP3, OGG, and WAV formats. |
Google Cloud Text to Speech API Example: A Practical Guide
Google Cloud offers a powerful Text-to-Speech API that can convert text input into natural-sounding speech. This service supports various languages and voices, giving developers the flexibility to integrate realistic voice synthesis into their applications. Whether you're building virtual assistants, audiobook generators, or voice-driven interfaces, the Text-to-Speech API is an essential tool for enhancing user experiences.
In this guide, we'll walk through the steps of using Google Cloud's Text-to-Speech API with a simple example. We'll cover the basics, including setting up your Google Cloud account, initializing the API client, and making a simple request to convert text into speech. You'll also learn how to fine-tune your output by adjusting parameters like voice selection and speaking rate.
Setting Up the Google Cloud Text-to-Speech API
- First, create a Google Cloud account if you don't already have one.
- Enable the Text-to-Speech API from the Google Cloud Console.
- Set up authentication by generating a service account key and downloading the JSON credentials file.
- Install the Google Cloud client library for Python (or any other supported language) using pip:
pip install google-cloud-texttospeech
Making Your First Request
Now that you have the necessary setup, let's make a basic request to convert text into speech.
from google.cloud import texttospeech
client = texttospeech.TextToSpeechClient()
synthesis_input = texttospeech.SynthesisInput(text="Hello, welcome to Google Cloud Text-to-Speech!")
voice = texttospeech.VoiceSelectionParams(
language_code="en-US",
ssml_gender=texttospeech.SsmlVoiceGender.FEMALE,
)
audio_config = texttospeech.AudioConfig(
audio_encoding=texttospeech.AudioEncoding.MP3
)
response = client.synthesize_speech(input=synthesis_input, voice=voice, audio_config=audio_config)
# Save the audio to a file
with open("output.mp3", "wb") as out:
out.write(response.audio_content)
Customizing the Output
The API offers several options to customize the speech output, such as adjusting the voice's pitch, speaking rate, and volume gain. Below is a brief overview of these options:
Option | Description |
---|---|
Pitch | Adjusts the pitch of the voice. Default is 0.0. Ranges from -20.0 to 20.0. |
Speaking Rate | Controls the speed at which the text is spoken. Default is 1.0. Values range from 0.25 to 4.0. |
Volume Gain | Adjusts the volume of the output audio. Default is 0.0. Ranges from -96.0 to 16.0. |
Note: Experiment with these parameters to achieve the desired speech output that fits your application's needs.
By following these steps, you'll be able to quickly integrate Google Cloud's Text-to-Speech capabilities into your projects, providing users with a more immersive experience through natural-sounding voice interactions.
Setting Up Google Cloud Text to Speech API on Your Account
To begin using Google Cloud Text to Speech API, you'll first need to configure your Google Cloud account and enable the necessary API services. This guide will walk you through the essential steps to set up everything correctly, ensuring you can integrate the speech synthesis capabilities into your projects without any hassle.
Follow the steps below to enable the Google Cloud Text to Speech API, set up authentication, and get started with the service. It's crucial to ensure that you have the necessary permissions and billing setup for smooth operation.
Steps to Enable the API
- Create a Google Cloud Account: If you haven't already, sign up for a Google Cloud account at https://cloud.google.com.
- Create a New Project: In the Google Cloud Console, create a new project or select an existing one.
- Enable the Text to Speech API: Navigate to the API & Services dashboard and enable the Text to Speech API by searching for it in the API library.
- Set up Billing: If not already done, set up billing for your Google Cloud project. Ensure you have an active billing account to avoid service disruptions.
Authentication Setup
Before making any API requests, you'll need to authenticate your application using a service account key. Follow these steps:
- Create a Service Account: Go to the "IAM & Admin" section and create a new service account. Assign it the "Project > Owner" role for full access.
- Generate a Key: Once the service account is created, generate a private key file in JSON format. This will be used for authentication.
- Set the Environment Variable: Download the JSON key file and set the GOOGLE_APPLICATION_CREDENTIALS environment variable to point to the file location.
Important: Ensure the service account has the necessary permissions to interact with the Text to Speech API. Always protect your service account keys and avoid exposing them publicly.
Testing the Setup
Once everything is set up, you can start making requests to the API. Here is a simple example of how to interact with the Text to Speech API using Python:
from google.cloud import texttospeech client = texttospeech.TextToSpeechClient() synthesis_input = texttospeech.SynthesisInput(text="Hello, world!") voice = texttospeech.VoiceSelectionParams(language_code="en-US", ssml_gender=texttospeech.SsmlVoiceGender.NEUTRAL) audio_config = texttospeech.AudioConfig(audio_encoding=texttospeech.AudioEncoding.MP3) response = client.synthesize_speech(input=synthesis_input, voice=voice, audio_config=audio_config) with open("output.mp3", "wb") as out: out.write(response.audio_content)
This will convert the text "Hello, world!" into an MP3 audio file, demonstrating that your setup is working properly.
Understanding Google Cloud Text to Speech API Pricing and Quotas
The Google Cloud Text to Speech API offers users the ability to convert text into natural-sounding speech using machine learning models. However, it’s important to understand the associated costs and limitations before integrating it into your applications. Pricing is structured based on the number of characters processed, with different rates for various voice models, and is influenced by factors such as usage volume and the type of voice selected.
Additionally, Google Cloud imposes specific quotas and usage limits to ensure fair usage of resources across all customers. This is particularly relevant for developers who plan to use the service heavily, as exceeding these limits may require additional configuration or a request for quota increases. Below are the key aspects of pricing and quotas that every user should be aware of when planning to use the API.
Pricing Breakdown
- Standard Voices: The pricing for standard voices is typically lower and charges are based on the number of characters processed.
- WaveNet Voices: WaveNet voices provide a more realistic and high-quality output but come at a higher cost per character.
- Free Tier: Users can benefit from a free tier that allows up to 4 million characters per month, which is ideal for testing or small-scale projects.
Quotas and Usage Limits
- Requests Per Minute: By default, the API allows a certain number of requests per minute to prevent excessive load on the system.
- Character Limits: The service has a daily limit for the total number of characters that can be processed. Exceeding this limit requires quota adjustment through the Google Cloud Console.
- Voice Type Restrictions: There are different quotas for Standard and WaveNet voices. WaveNet voices generally have stricter limitations due to their higher processing cost.
Important: It’s crucial to monitor usage and manage quotas regularly, as exceeding limits may result in service interruptions or unexpected charges. Always check the Google Cloud Console for up-to-date information on your API usage.
Pricing Table
Voice Type | Cost per Character | Free Tier |
---|---|---|
Standard Voices | $4.00 per 1 million characters | Up to 4 million characters/month |
WaveNet Voices | $16.00 per 1 million characters | Up to 1 million characters/month |
Configuring Audio Output Options for Your Application
When integrating speech synthesis into your application using the Google Cloud Text-to-Speech API, it is essential to properly configure the audio output settings to ensure compatibility with your use case. The API provides a variety of options that control the audio format, quality, and other parameters necessary for creating the desired output.
Adjusting these parameters allows developers to fine-tune the speech output, whether it's for real-time interaction, audio file generation, or specialized audio devices. This flexibility enables customization in both the technical and user experience aspects of your application.
Audio Format Options
The API supports several audio formats to meet the needs of different platforms and devices. Below are the main options available:
- MP3: A widely used compressed audio format suitable for low bandwidth or storage requirements.
- OGG_OPUS: A high-quality audio format that provides excellent speech clarity while keeping the file size small.
- LINEAR16: A raw audio format that provides uncompressed output, ideal for high-fidelity requirements.
Setting Audio Encoding
To choose the audio encoding format, you need to specify it within the request parameters:
- Select the encoding type based on your platform’s requirements.
- Ensure that the output format aligns with your application’s performance needs.
- Adjust bitrate settings if necessary, depending on the chosen encoding format.
Tip: For applications with strict latency requirements, using OGG_OPUS may offer better performance compared to MP3, which requires more processing time.
Additional Configuration Options
Further customization can be done through the API's audio configuration settings:
Parameter | Description |
---|---|
speakingRate | Adjust the speed of the speech output, ranging from 0.25 (slower) to 4.0 (faster). |
pitch | Modify the pitch of the voice, where 0 is the default pitch, negative values lower the pitch, and positive values increase it. |
volumeGainDb | Adjust the output volume in decibels, with values from -96.0 to 16.0 dB. |
Integrating Google Cloud Text to Speech with Your Web or Mobile App
Integrating Google Cloud Text to Speech API into your web or mobile application allows you to convert text into natural-sounding speech, enhancing accessibility and user experience. With its ability to synthesize speech in multiple languages and voices, you can cater to a diverse range of users. The process of adding this feature is straightforward, thanks to the robust APIs provided by Google Cloud.
To integrate the Text to Speech API, you need to perform a series of steps such as setting up a Google Cloud project, enabling the necessary API, and integrating the required SDK into your app. Once these steps are completed, you can begin using the API to convert text into speech and customize the output according to your needs.
Steps for Integration
- Create a Google Cloud Project
- Visit the Google Cloud Console.
- Create a new project or select an existing one.
- Enable billing for your project.
- Enable the API
- In the Google Cloud Console, navigate to the API Library.
- Search for “Text to Speech API” and enable it for your project.
- Authenticate Requests
- Create a service account and download the authentication key.
- Set up the environment variable for authentication in your app.
- Install SDK and Libraries
- Install the client library for your programming language (e.g., Python, Node.js, Java).
Important: Make sure that the Google Cloud API credentials are securely stored to avoid unauthorized access to your services.
Customizing the Voice Output
Once the integration is complete, you can adjust the speech output to match your requirements. Google Cloud Text to Speech offers various options for customizing the voice, including:
- Voice Selection: Choose from different voices (male, female, or neutral) and languages.
- Speech Rate: Adjust the speed at which the text is spoken.
- Pitch Control: Modify the pitch to make the voice sound more natural or formal.
- Audio Format: Select from various audio formats such as MP3, OGG, or WAV.
Example Output Table
Parameter | Value |
---|---|
Voice | en-US-Wavenet-D (Male) |
Speech Rate | 1.2x (Normal) |
Pitch | 0 (Default) |
Audio Format | MP3 |
How to Choose the Right Voice Model for Your Project
When working with speech synthesis services like Google Cloud's Text-to-Speech API, selecting the correct voice model is a crucial step. The model you choose will have a direct impact on the quality and naturalness of the generated speech, as well as its overall effectiveness in meeting your project’s specific needs. Understanding the different types of voice models available is essential for achieving optimal results, whether you're developing a virtual assistant, creating content for an audiobook, or generating automated responses for customer support systems.
Each voice model offers distinct advantages and limitations based on factors like language support, accent, expressiveness, and computational resource requirements. Below, we’ll explore the key criteria you should consider when making your choice.
Key Factors in Choosing a Voice Model
To make an informed decision, consider the following factors:
- Language and Accent: Choose a voice model that supports the language and accent of your target audience. Some models are tailored to specific regions and can have more natural-sounding accents for localized speech.
- Voice Type: Decide whether you need a male, female, or gender-neutral voice. Some projects may benefit from a specific tone or persona, such as a more authoritative or friendly voice.
- Expressiveness: Some models support a wide range of emotions, such as joy, sadness, or enthusiasm, while others are more neutral. If your project requires dynamic expression, opt for a model that supports prosody and emotional variability.
Model Types Available
Google Cloud offers two primary voice model categories:
- Standard Voice Models: These models are optimized for efficiency and deliver reliable, clear speech but might lack the nuanced expressiveness of more advanced options.
- WaveNet Voice Models: These models offer superior naturalness and prosody, making them ideal for applications that prioritize high-quality, lifelike speech synthesis.
Performance and Use Case Suitability
The choice between Standard and WaveNet models largely depends on your project’s specific needs:
Criteria | Standard Model | WaveNet Model |
---|---|---|
Voice Quality | Clear, but robotic | Natural and expressive |
Latency | Faster | Slower |
Cost | Lower | Higher |
Tip: If latency is a concern and the naturalness of the voice is secondary, consider opting for Standard models. For projects that require a more lifelike, human-like voice, WaveNet models are recommended, despite the higher cost and slower response time.
Handling Errors and Debugging Common Problems
When working with the Google Cloud Text-to-Speech API, it's important to be prepared for errors that may arise during integration. These errors can range from authentication issues to problems with the request format. Identifying and resolving these errors quickly will ensure smooth interaction with the API.
Common issues often relate to invalid API keys, incorrect request formats, or limitations in quotas. By using the right tools and following best practices for error handling, developers can ensure that their integration works as expected and that problems are addressed efficiently.
Common Error Responses
- Invalid API Key: This error occurs when the API key provided in the request is missing, incorrect, or expired.
- Quota Exceeded: When the usage limit for the API is reached, requests will be rejected until the quota is reset.
- Malformed Request: If the request body does not follow the required structure, a 400 error will be returned.
- Unauthorized Access: This happens when the authentication token is invalid or when the user does not have the correct permissions to access the resource.
Debugging Steps
- Check the API key for correctness and ensure it is included in the request header.
- Verify the request body format. Ensure that parameters like "input" and "voice" are correctly specified.
- Inspect API quota usage in the Google Cloud Console. If limits are exceeded, adjust usage or request a quota increase.
- Examine the response code and error message returned by the API to pinpoint the exact issue.
Helpful Tools for Troubleshooting
For detailed error information, always refer to the response body. It typically contains an error message and a code that helps identify the issue.
Example Error Response
Error Code | Message |
---|---|
400 | Invalid request format. Please check the API documentation for correct syntax. |
401 | Unauthorized. Please check your API key and permissions. |
403 | Quota exceeded. Please check your usage limits in the Google Cloud Console. |
Best Practices for Optimizing Text to Speech Performance
When working with text-to-speech systems, especially when utilizing cloud APIs, it is crucial to ensure that the performance is maximized both in terms of speed and quality. Optimizing the way the API processes input can significantly reduce latency and increase the overall user experience. Here are some essential tips and techniques that can help in achieving better performance when using text-to-speech services.
By focusing on several core areas such as audio file format, proper request configuration, and effective resource management, users can prevent issues related to unnecessary delays or suboptimal sound quality. Adopting best practices can result in faster, more accurate speech synthesis and improved efficiency of the entire workflow.
Key Optimization Strategies
- Use Efficient Audio Formats: Audio file formats such as MP3 or OGG are often preferred for their smaller size and faster download speeds compared to formats like WAV. This helps reduce latency and improves playback speed without sacrificing quality.
- Preprocess Text Inputs: Before sending text to the API, clean up the text by removing unnecessary punctuation, abbreviations, and irrelevant words. This will ensure that the synthesis is faster and more accurate.
- Choose the Right Voice Model: Selecting the most suitable voice model based on the language and accent of your target audience can improve both the accuracy and clarity of the generated speech.
Optimizing Request Configuration
- Batch Requests: Instead of sending single text strings to the API, batch multiple requests into one to reduce overhead and minimize the number of API calls.
- Adjust Speaking Rate and Pitch: Fine-tuning the speaking rate and pitch settings can help balance performance with natural-sounding speech. Test these parameters to find the best combination for your application.
- Use Streaming for Real-Time Applications: For real-time or interactive applications, consider using streaming API requests to minimize the delay between sending the text and receiving the spoken output.
Tip: Always test different configurations to determine the optimal settings for your specific use case. Performance can vary based on factors such as text length, network conditions, and voice model.
Resource Management
Optimizing the use of resources like memory and processing power is essential for achieving the best results. Ensuring that your API calls are efficient and do not overload the system can contribute significantly to performance.
Resource | Best Practice |
---|---|
Memory | Minimize the memory usage by avoiding excessively long text inputs and using compressed audio formats. |
CPU | Distribute the workload evenly to prevent any bottlenecks that could lead to slower processing times. |