R Create a Vector of Ones
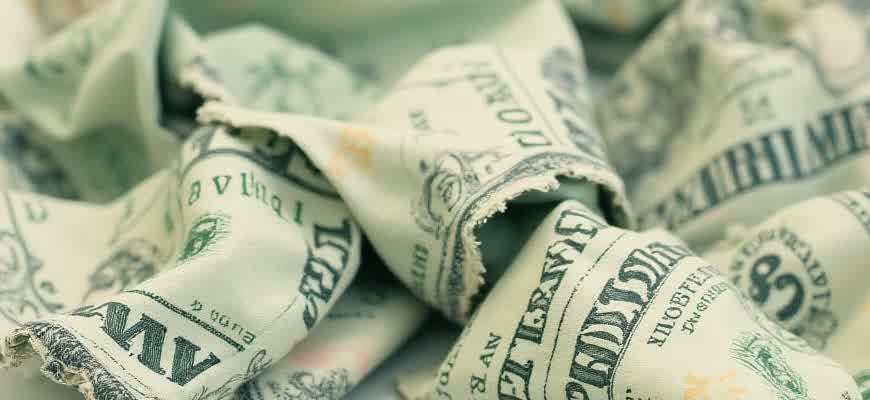
In R, vectors are a fundamental data structure, and there are various ways to initialize them. One of the most common tasks is creating a vector filled with ones. This can be done using the rep() function or the numeric() function with an assignment of values. Below are the primary methods to achieve this.
- Using the rep() function: The
rep()
function repeats elements to form a vector. For example,rep(1, 5)
creates a vector of length 5, where all elements are 1. - Using the numeric() function: The
numeric()
function initializes a numeric vector of a specified length, and with a simple assignment, we can populate it with ones.
Let's take a look at a simple example of both approaches:
Method | Code | Result |
---|---|---|
rep() function | rep(1, 5) |
[1] 1 1 1 1 1 |
numeric() function | ones <- numeric(5); ones[] <- 1 |
[1] 1 1 1 1 1 |
The rep() function is useful for repeating a specified value multiple times, while numeric() initializes a numeric vector, allowing you to later assign values.
Step-by-Step Guide to Creating a Vector of Ones in R
In R, a vector of ones can be created easily using the `rep()` or `numeric()` functions. Vectors are essential data structures in R, and often you may need to generate a sequence of ones for various tasks like mathematical operations or initializing matrices. Below is a guide on how to create a vector filled with ones in R, using different approaches.
This step-by-step guide demonstrates a few methods for creating a vector of ones, each with its specific use case. Understanding these methods will help you choose the best approach based on your needs, whether it's for matrix initialization or iterative calculations.
Method 1: Using the `rep()` Function
The `rep()` function is versatile and can repeat any value for a specified number of times. To create a vector of ones, we simply specify `1` as the value to repeat and provide the length of the desired vector.
- Use `rep(1, times = n)`, where `n` is the number of ones you need in the vector.
- For example, to create a vector with 5 ones, use `rep(1, times = 5)`.
Example:rep(1, times = 5)
will generate a vector:1 1 1 1 1
Method 2: Using the `numeric()` Function
The `numeric()` function creates a vector of zeros, but we can modify the result by adding one to all the elements in the vector. This is useful when you need to initialize vectors with a specific number of elements, where you may later update the values.
- First, create a zero-filled vector with `numeric(n)`.
- Then, add `1` to each element to make all values ones.
Example:numeric(5) + 1
will generate a vector:1 1 1 1 1
Comparison Table
Method | Code Example | Output |
---|---|---|
Using rep() | rep(1, times = 5) |
1 1 1 1 1 |
Using numeric() and addition | numeric(5) + 1 |
1 1 1 1 1 |
Both methods are straightforward, but depending on the context, one may be more suitable than the other. The `rep()` function is ideal for quick vector creation, while the `numeric()` function offers a good starting point for vectors that may require further manipulation.
How to Define the Size of a Vector of Ones in R
In R, vectors are fundamental structures that store multiple values of the same type. When you need a vector that consists entirely of ones, you can easily create it using the `rep()` function or the `numeric()` function. The length of this vector can be defined by specifying the number of elements it should contain. Defining the size allows you to create vectors of varying lengths, depending on the needs of your analysis.
The most common method to generate a vector of ones is using the `rep()` function, which repeats a given value. By specifying the number of repetitions, you control the size of the resulting vector. Here, we will explore different ways to define the vector's length and how this affects the output.
Methods to Define the Vector Length
- Using rep() function: The `rep()` function repeats the number 1 for the specified number of times.
- Using numeric() function: The `numeric()` function initializes a numeric vector with a specified length, and by setting all elements to 1, you create a vector of ones.
- Using a loop: A loop can also be used to populate a vector of ones, but it is generally less efficient than the built-in functions.
Note: The most efficient and commonly used approach is through the `rep()` function, as it directly creates the desired vector without unnecessary computations.
Example Code Snippets
- Using `rep()`:
ones_vector <- rep(1, 5)
This creates a vector of 5 ones: 1 1 1 1 1
- Using `numeric()`:
ones_vector <- numeric(5)
This initializes a vector of length 5, and by assigning 1 to each element:
ones_vector[] <- 1
Results in: 1 1 1 1 1
Comparison of Methods
Method | Code Example | Result |
---|---|---|
rep() | rep(1, 5) |
1 1 1 1 1 |
numeric() + Assignment | ones_vector <- numeric(5) ones_vector[] <- 1 |
1 1 1 1 1 |
How to Integrate a Vector of Ones with Other R Functions for Complex Data Operations
R offers an efficient way to handle matrices, data frames, and vectors. A vector of ones can be a fundamental component when performing various data manipulation tasks. By combining this vector with other functions, users can generate advanced operations to simplify complex calculations or matrix manipulations. Let’s explore how you can leverage this in different contexts.
The key idea is to use the vector of ones in tandem with functions such as apply(), rep(), or matrix operations like cbind() and rbind(). These combinations allow for the manipulation and transformation of data in an organized way, whether you’re scaling matrices or modifying the structure of data frames.
Combining Ones Vector with Functions
- Element-wise Operations: The ones vector can be used for element-wise arithmetic when combined with other numeric vectors or matrices.
- Matrix Operations: Create matrices with ones for initial values and adjust them as necessary using functions like cbind() and rbind().
- Replicating Values: Use the rep() function to replicate the vector of ones across multiple columns or rows, depending on the structure you need.
Example Use Cases
- Creating a constant column of ones in a data frame for regression models.
- Building identity matrices by multiplying the ones vector with a scalar value.
- Adjusting the output of apply() or lapply() to perform operations across subsets of data.
By integrating a vector of ones with R functions, you can efficiently manipulate your data and perform tasks such as matrix scaling or modifying entire datasets based on a specific constant value.
Example Table: Matrix Operations with Ones Vector
Operation | Code Example | Result |
---|---|---|
Creating a matrix of ones | matrix(rep(1, 9), nrow=3, ncol=3) | A 3x3 matrix with all values equal to 1 |
Adding ones vector to a matrix | cbind(matrix(1:3, nrow=3), rep(1, 3)) | A matrix with an additional column of ones |
Multiplying with a scalar | rep(1, 5) * 10 | A vector [10, 10, 10, 10, 10] |
Common Pitfalls When Creating a Vector of Ones in R and How to Avoid Them
Creating a vector filled with ones in R is a simple task, but there are some common mistakes users may encounter, especially when dealing with large datasets or when performing specific operations on the vector. Understanding these pitfalls can save time and reduce errors in data processing tasks. Here are some of the most frequent challenges faced by R users when generating a vector of ones and how to avoid them.
R provides several methods for constructing vectors of ones, such as using the rep()
function, ones()
from certain packages, or the numeric()
function. Despite the simplicity of these approaches, users often face confusion over unexpected behavior, which can lead to bugs or incorrect outputs. Below are a few important issues to be aware of.
1. Incorrect Vector Length
One of the most common mistakes is specifying the wrong vector length. Users may accidentally create a vector of the wrong size by misusing the length
argument or not properly defining the size in their function calls. For example, calling rep(1, "5")
will result in an error because the second argument must be numeric. Here’s how to avoid this issue:
- Always double-check the argument passed to the length parameter, ensuring it's an integer.
- Use functions that accept numeric values directly, like
rep(1, 5)
, to create a vector of ones of length 5.
Tip: To avoid confusion, always assign the length explicitly and avoid using non-numeric values as the second argument in functions like
rep()
.
2. Confusion with Initialization Methods
There are multiple ways to initialize a vector of ones, and choosing the wrong method can lead to unexpected results. For example, using the numeric()
function will create a vector of zeros, not ones. Another issue arises when using the rep()
function improperly, such as trying to use it with incorrect data types or forgetting to specify the number of repetitions.
- Correct method:
rep(1, 5)
creates a vector of five ones. - Incorrect method:
numeric(5)
creates a vector of five zeros, not ones.
Reminder: Always verify that the function you're using is intended for creating vectors with ones and not initializing them with zeros or other values.
3. Misunderstanding of Vector Types
Another pitfall occurs when the data type of the vector is not properly considered. For instance, users might expect a vector of integers when they create a vector of ones, but it might actually create a numeric vector with floating-point numbers (1.0). This could lead to issues if integer precision is required in further calculations.
Method | Expected Output | Resulting Data Type |
---|---|---|
rep(1, 5) |
1, 1, 1, 1, 1 | Numeric |
as.integer(rep(1, 5)) |
1, 1, 1, 1, 1 | Integer |
To avoid this issue, be sure to use the as.integer()
function if you need an integer vector, as shown above.
Optimizing Memory Usage with Large Vectors of Ones in R
When dealing with large datasets in R, efficiently managing memory usage becomes crucial, especially when constructing vectors of ones. These vectors can consume a significant amount of memory, particularly when their length is large. Understanding the underlying data structures and techniques to minimize memory consumption is key for handling large-scale computations and ensuring that the system operates smoothly.
One of the primary challenges when creating vectors filled with ones in R is that, by default, R stores each element as a separate entity. This can be inefficient, as a vector of ones does not require the actual storage of each element. Instead, it would be more memory-efficient to represent the vector in a compressed form. Below are strategies to optimize memory when working with large vectors of ones.
Methods to Optimize Memory Usage
- Use of Sparse Matrices: Instead of creating a traditional vector of ones, consider using sparse matrices. The Matrix package in R allows for efficient storage of vectors and matrices with a majority of zero elements.
- Integer Representation: Using the
integer()
function can create a zero-filled vector with lower memory usage. For vectors with a majority of ones, it's often more efficient to use a logical vector withTRUE
values. - Recycling and Compression: In certain scenarios, you can take advantage of vector recycling and compression techniques to avoid storing unnecessary repetitions of ones.
Memory Consumption Comparison
Method | Memory Usage | Time Complexity |
---|---|---|
Sparse Matrix | Low | O(1) for creation, O(n) for operations |
Logical Vector (TRUE) | Moderate | O(n) |
Integer Vector | Low | O(n) |
Note: Sparse matrices are particularly useful when most of the elements are zero. However, if the vector is dense (mostly ones), a logical or integer vector might be a better choice for memory optimization.
How to Utilize a Vector of Ones in R for Matrix Operations and Mathematical Modeling
A vector filled with ones plays a significant role in mathematical modeling and matrix operations, particularly in the context of linear algebra and simulations. In R, creating a vector of ones is straightforward using the function rep(1, n), where n specifies the length of the vector. This vector can serve various purposes such as representing constant values in a system of equations or performing element-wise multiplication and addition with other matrices.
When working with matrix operations, a vector of ones can be useful in the construction of identity matrices, solving systems of linear equations, or performing matrix transformations. Additionally, in optimization problems, vectors of ones can act as coefficients or constants in objective functions, helping to model real-world problems like resource allocation or financial analysis.
Applications in Matrix Operations
- Creating matrix identities: A vector of ones can be used as a building block for generating matrices where each element is a constant or when calculating a matrix sum with uniform contributions.
- Vector-matrix multiplication: Multiplying a vector of ones by a matrix gives the row sums, which can be helpful in data aggregation or feature engineering.
- Simulating uniform inputs: In simulations where each entity has equal weight or contribution, a vector of ones can be used to initialize the system.
Example of Mathematical Modeling with Vectors of Ones
- In a simple optimization model, you might create a vector of ones to represent constraints or equality conditions across multiple variables.
- Using the vector in matrix multiplication: Multiply a vector of ones by a data matrix to compute the sum of each row or column, useful in statistical aggregation.
- Solving equations: A vector of ones could be used to create a system where all coefficients are equal, simplifying certain types of linear problems.
Vectors filled with ones are often used in mathematical modeling to create consistency across all variables, which can simplify the problem-solving process or reduce the complexity of calculations.
Table: Example of Vector of Ones in Matrix Multiplication
Matrix A | Vector of Ones | Resulting Vector |
---|---|---|
1 2 3 | 1 | 6 |
4 5 6 | 1 | 15 |
7 8 9 | 1 | 24 |